 |
 |
Purpose
- Understand how references work
- Gain more experience using objects
There are two files that must be submitted,
UsingReferences.java
and EmailHarvester.java.
Remember that if you don't finish during the lab time, that's fine -- you
need to
submit the files you have done so far, get a
lab
extension, and then complete (and submit) the lab within the next 24 hours.
Lastly, this lab may be a bit challenging. If you are unsure about any part of it, ask a TA -- that's why they are there!
Using References
- For this part, you will have to enter a number of answers as comments in
the program file. We are looking for short comments here -- concise,
and to the point. You don't need to spend a lot of time writing essay
answers.
- Start by saving the UsingReferences.java
file to your home directory (right-click and select "save as...").
- Consider the following code:
String s;
String t = null;
String u = "";
System.out.println (t);
System.out.println (u);
What does memory look like after this code completes (i.e. what do the String references point to)?
And what is the difference between the three lines? Enter your
answers as a comment in the UsingReferences.java file (just text, no diagrams
necessary).
- Copy this code into UsingReferences.java (under the comment that says
'first code segment goes here'). To save you the time of retyping in
the code, you can highlight the above code with the mouse, select
Edit->Copy, then in JCreator select Edit->Paste. Compile and run the
program. Did the results match what you expected? If not, figure
out why, and/or ask a TA for help.
- Consider the following code:
s = "string1";
t = "string2";
u = s;
s = t;
t = u;
System.out.println (s);
System.out.println (t);
System.out.println (u);
What does memory look like after this code completes (i.e. what do the String references point to)? Enter your answers as comments in
the UsingReferences.java file (just text, no diagrams necessary).
- Copy this code into UsingReferences.java (under the comment that says
'second code segment goes here'). Compile and run the program.
Did the results match what you expected? If not, figure out why,
and/or ask a TA for help.
- Consider the following code:
s = "string1";
t = null;
s = t;
System.out.println (s);
System.out.println (t);
What does memory look like after this code completes (i.e. what do the String references point to)? Enter your
answer as a comment in the UsingReferences.java file (just text, no diagrams
necessary).
- Copy this code into UsingReferences.java (under the comment that says
'third code segment goes here'). Compile and run the program.
Did the results match what you expected? If not, figure out why,
and/or ask a TA for help.
- Consider the following code:
s = null;
System.out.println (s.length());
What does memory look like after this code completes (i.e. what do the String references point to)? Enter your
answer as a comment in the UsingReferences.java file (just text, no diagrams
necessary).
- Copy this code into UsingReferences.java (under the comment that says
'fourth code segment goes here'). Compile and run the program.
Did the results match what you expected? Did the program work
correctly?
If not, figure out why, and/or ask a TA for help.
- Fix the program so that it runs without an error. You can do any
fix so that the last code segment works.
- When you are finished with the UsingReferences.java,
submit it.
Using Objects
- For this part of the lab, you are going to develop a program that extracts
e-mail addresses. This is similar to the e-mail harvester program, found
on pages 134-136 of your textbook. Indeed, steps 1-6 below correspond to
the steps in the textbook (we've added steps 0 and 7).
- For this program, the user will enter a entire line of text, such as:
Write the authors at javaprogramdesigne@mhhe.com some day.
- Note that the program assumes that the e-mail address is within an entire sentence - i.e., that there is a space both before and after it. So when you test your program, make sure you have a word or two both before and after the e-mail address.
- The program must extract the e-mail address (javaprogramdesigne@mhhe.com)
from the passed in String. Note that the string may have a number of
words both before and after the e-mail address. You can assume that
the text the user enters will have one and only one '@' character.
- Start by saving the EmailHarvester.java
file to your home directory (right-click and select "save as...").
- The program has a series of steps that you must complete, and a few that have been done for you already.
- Step 0: Display program legend. This should be familiar, and just
displays a message stating what the program does. This step has already been completed for you.
- Step 1: Prompt for input text message. This just prints a message
telling the user to enter a line of text that contains an e-mail address. This step has already been completed for you.
- Step 2: Get input text message. You will have to initialize a
Scanner object here, and then use that Scanner object to obtain the input
from the keyboard. Note that you want to use the nextLine() method,
as that will get the entire line -- and all the words and spaces therein. This step has already been completed for you.
- Step 3: Find the index of the '@' character in the message. This
is via the indexOf(str) method, which you used in the last lab. More information about this method can be
found in the
documentation for the String class. Save this value as an int
variable named j, and print it out to the screen.
- Step 4: Find the index of the space at the beginning of the e-mail
address. This is done via the lastIndexOf(str, fromIndex) method, which we have not
gone over in class (details of which can be found in the
documentation for the String class). Essentially, you want to search backwards (via lastIndexOf()) from the '@' character (you know the position of the '@' from the last step) to find the space at the beginning of the e-mail address. Save this value as an int variable
i, and print it out to the screen.
- Step 5: Find the index of the space at the end of the e-mail address.
This is done via the indexOf(str, fromIndex) method (details of which can be found in the
documentation for the String class). Save this value as an int
variable k, and print it out to the screen.
- Step 6: Set the e-mail address to the String from i to k. This is
done via the substring() method. Save it into an
appropriately named String object (you pick the name).
- Step 7: Print out the e-mail address. This just prints out the
e-mail address from step 6.
- When you are finished with the EmailHarvester.java,
submit it.
There are many situations where one would like to copy the program output
(in the black text window) into their Java code as a comment. In
particular, this is a convenient way to show that you tested a given
program. This part of the lab
will go over an easy way to do this.
- Run E-mail harvester, and enter a sentence with your e-mail address.
The window should now say, "Press any key to continue..."
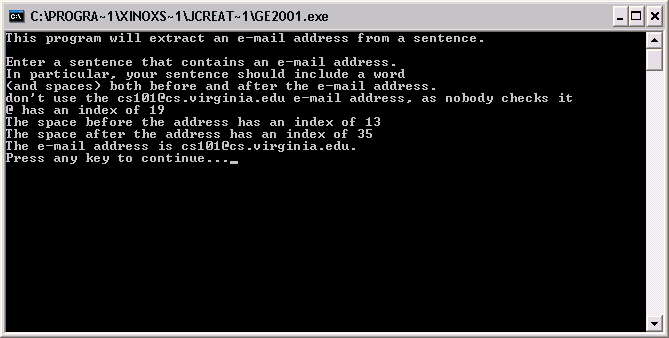
- From the menu in the upper-left (click on the '
'
icon), select Edit->Select All.
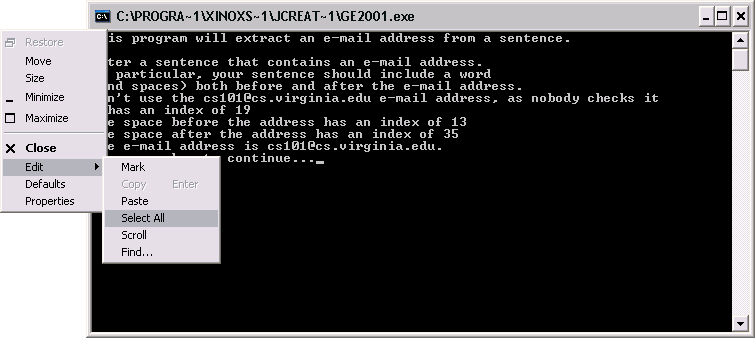
- This will automatically highlight all the text in the window.
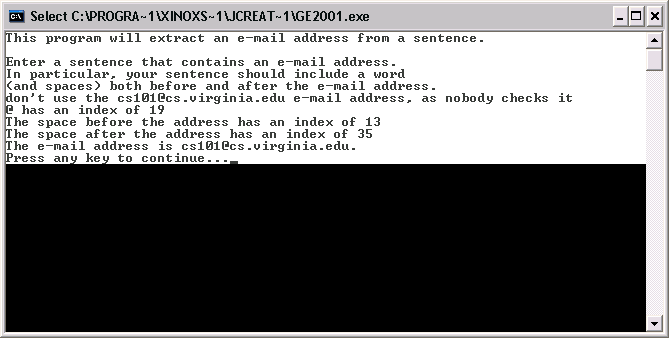
- You can then hit Enter, which will copy the text to the clipboard,
so you can use it in the next step. Hitting enter will also cause
the highlighting will disappear.
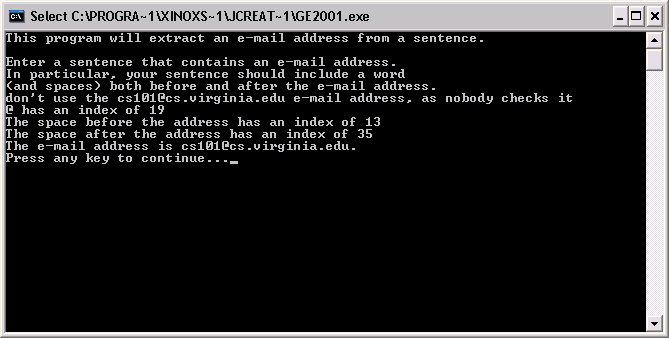
- Now switch back to JCreator, select an appropriate place to enter
the output, and select Edit->Paste (or hit Control-V).
- You should now comment out the output in JCreator, so that it will
compile properly.
- Compile the code.
-
Re-submit EmailHarvester.java.
Work on the homework
Use any remaining time to work on
homework J1,
and homework C1.
|
 |